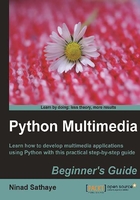
Time for action – capture screenshots at intervals
Imagine that you are developing an application, where, after certain time interval, the program needs to automatically capture the whole screen or a part of the screen. Let's develop code that achieves this.
- Write the following code in a Python source file. When the code is executed, it will capture part of the screen after every two seconds. The code will run for about three seconds.
1 import ImageGrab 2 import time 3 startTime = time.clock() 4 print "\n The start time is %s sec" % startTime 5 # Define the four corners of the bounding box. 6 # (in pixels) 7 left = 150 8 upper = 200 9 right = 900 10 lower = 700 11 bbox = (left, upper, right, lower) 12 13 while time.clock() < 3: 14 print " \n Capturing screen at time %.4f sec" \ 15 %time.clock() 16 screenShot = ImageGrab.grab(bbox) 17 name = str("%.2f"%time.clock())+ "sec.png" 18 screenShot.save("C:\\images\\output\\" + name) 19 time.sleep(2)
- We will now review the important aspects of this code. First, import the necessary modules. The
time.clock()
keeps track of the time spent. On line 11, a bounding box is defined. It is a4-tuple
that defines the boundaries of a rectangular region. The elements in thistuple
are specified in pixels. In PIL, the origin (0, 0) is defined in the top-left corner of an image. The next illustration is a representation of a bounding box for image cropping; see how left, upper and right, lower are specified as the ends of a diagonal of rectangle.Example of a bounding box used for image cropping.
- The
while
loop runs till thetime.clock()
reaches three seconds. Inside the loop, the part of the screen bounded withinbbox
is captured (see line 16) and then the image is saved on line 18. The image name corresponds to the time at which it is taken. - The
time.sleep(2)
call suspends the execution of the application for two seconds. This ensures that it grabs the screen every two seconds. The loop repeats until the given time is reached. - In this example, it will capture two screenshots, one when it enters the loop for the first time and the next after a two-second time interval. In the following illustration, the two images grabbed by the code are shown. Notice the time and console prints in these images.
The preceding screenshot is taken at time 00:02:15 as shown dialog. The next screenshot is taken after 2 seconds, at wall clock time, 00:02:17.
What just happened?
In the preceding example, we wrote a simple application that captures the screen at regular time intervals. This helped us to learn how to grab a screen region using ImageGrab
.
Cropping
In previous section, we learned how to grab a part of the screen with ImageGrab
. Cropping is a very similar operation performed on an image. It allows you to modify a region within an image.