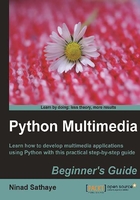
Time for action – flipping
Imagine that you are building a symmetric image using a bunch of basic shapes. To create such an image, an operation that can flip (or mirror) the image would come in handy. So let's see how image flipping can be accomplished.
- Write the following code in a Python source file.
1 import Image 2 inPath = "C:\\images\\Flip.png" 3 img = Image.open(inPath) 4 outPath = "C:\\images\\Flip_out.png" 5 foo = img.transpose(Image.FLIP_LEFT_RIGHT) 6 foo.save(outPath)
- In this code, the image is flipped horizontally by calling the
transpose
method. To flip the image vertically, replace line 5 in the code with the following:foo = img.transpose(Image.FLIP_TOP_BOTTOM)
- The following illustration shows the output of the preceding code when the image is flipped horizontally and vertically.
- The same effect can be achieved using the
ImageOps
module. To flip the image horizontally, useImageOps.mirror
, and to flip the image vertically, useImageOps.flip
.import ImageOps # Flip image horizontally foo1 = ImageOps.mirror(img) # Flip image vertically foo2 = ImageOps.flip(img)
What just happened?
With the help of example, we learned how to flip an image horizontally or vertically using Image.transpose
and also by using methods in class ImageOps
. This operation will be applied later in this book for further image processing such as preparing composite images.
Capturing screenshots
How do you capture the desktop screen or a part of it using Python? There is ImageGrab
module in PIL. This simple line of code will capture the whole screen.
img = ImageGrab.grab()
Where, img
is an instance of class Image
.
However, note that in PIL Version 1.1.6, the ImageGrab
module supports screen grabbing only for Windows platform.