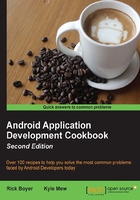
Creating a widget at runtime
As mentioned before, generally, the UI is declared in XML
files and then modified during runtime through the Java code. It is possible to create the UI completely in Java code, though for a complex layout, it would generally not be considered best practice.
The GridView example from the previous chapter was created in code. But unlike the GridView recipe, in this recipe, we are going to add a view to the existing layout defined in activity_main.xml
.
Getting ready
Create a new project in Android Studio and call it RuntimeWidget
. Select the Empty Activity option when prompted for the Activity type.
How to do it...
We will start by adding an ID attribute to the existing layout so we can access the layout in code. Once we have a reference to the layout in code, we can add new views to the existing layout. Here are the steps:
- Open the
res/layout/activity_main.xml
and add an ID attribute to the mainRelativeLayout
, as follows:android:id="@+id/layout"
- Completely remove the default
<TextView>
element. - Open the
MainActivity.java
file so we can add code to theonCreate()
method. Add the following code (aftersetContentView())
to get a reference to theRelativeLayout
:RelativeLayout layout = (RelativeLayout)findViewById(R.id.layout);
- Create a DatePicker and add it to the layout with the following code:
DatePicker datePicker = new DatePicker(this); layout.addView(datePicker);
- Run the program on a device or emulator.
How it works...
This is hopefully very straightforward code. First, we get a reference to the parent layout using findViewById
. We added the ID to the existing RelativeLayout (in step 1) to make it easier to reference. We create a DatePicker in code and add it to the layout with the addView()
method.
There's more...
What if we wanted to create the entire layout from code? Though it may not be considered best practice, there are times when it is certainly easier (and less complex) to create a layout from code. Let's see how this example would look if we didn't use the layout from activity_main.xml
. Here's how the onCreate() would look:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); RelativeLayout layout = new RelativeLayout(this); DatePicker datePicker = new DatePicker(this); layout.addView(datePicker); setContentView(layout); }
In this example, it's really not that different. If you create a view in code and want to reference it later, you either need to keep a reference to the object, or assign the view an ID to use findViewByID()
. To give a view an ID, use the setID() method by passing in View.generateViewId() (to generate a unique ID) or define the ID using <resources> in xml.