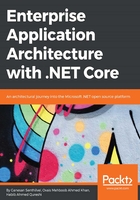
上QQ阅读APP看书,第一时间看更新
Introducing dependency injection
Dependency Injection design pattern fulfills the dependency inversion principle of the SOLID design principles. There are three main forms of dependency injection:
- Constructor injection: An example of this is shown in the DIP section
- Setter injection: Let's look at an example code for setter injection:
public class OrderProcessorWithSetter : IOrderProcessor
{
private IOrderRepository _orderRepository;
private IOrderNotifier _orderNotifier;
public IOrderRepository Repository
{
get { return _orderRepository; }
set { _orderRepository = value; }
}
public IOrderNotifier Notifier
{
get { return _orderNotifier; }
set { _orderNotifier = value; }
}
public void Process(IOrder order)
{
//Perform validations..
if (_orderRepository.Save(order))
_orderNotifier.Notify(order);
}
}
Now we look at an example client code using the setter injection:
[Fact]
public void Test_DI_With_Setter()
{
var someOrder = new DIP.Order();
var op = new DIP.Good.OrderProcessorWithSetter();
op.Repository = new DIP.OrderRepository();
op.Notifier = new DIP.OrderNotifier();
op.Process(someOrder);
}
- Interface injection: Let's take a look at an example code for interface injection. The following are the two interfaces that will be used for our sample injection:
public interface InjectOrderRepository
{
void SetRepository(IOrderRepository orderRepository);
}
public interface InjectOrderNotifier
{
void SetNotifier(IOrderNotifier orderNotifier);
}
And here is the OrderProcessorWithInterface class that implements the interfaces that are used for injection (usually via a DI framework):
public class OrderProcessorWithInterface : InjectOrderRepository,
InjectOrderNotifier, IOrderProcessor
{
private IOrderRepository _orderRepository;
private IOrderNotifier _orderNotifier;
public void SetRepository(IOrderRepository orderRepository)
{
_orderRepository = orderRepository;
}
public void SetNotifier(IOrderNotifier orderNotifier)
{
_orderNotifier = orderNotifier;
}
public void Process(IOrder order)
{
//Perform validations..
if (_orderRepository.Save(order))
_orderNotifier.Notify(order);
}
}
Let's have a look at the client code that uses the simple example of interface injection:
[Fact]
public void Test_DI_With_Interface()
{
var someOrder = new DIP.Order();
var op = new DIP.Good.OrderProcessorWithInterface();
//Creation of objects and their respective dependencies
(components/services inside) are usually done by the
DI Framework
op.SetRepository(new DIP.OrderRepository());
op.SetNotifier(new DIP.OrderNotifier());
op.Process(someOrder);
}