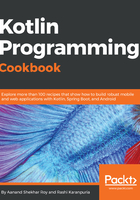
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's go through the given steps to create an idiomatic logger in Kotlin:
- First, let's see how it was done in Java. In Java, SLF4J is used and considered de-facto, so much that logging seems like a solved problem in Java language. Here's what a Java implementation would look like:
private static final Logger logger = LoggerFactory.getLogger(CurrentClass.class);
…
logger.info(“Hi, {}”, name);
- It also works fine with Kotlin, obviously with minor modifications:
val logger = LoggerFactory.getLogger(CurrentClass::class)
…
logger.info(“Hi, {}”, name)
However, apart from this, we can utilize the power of Kotlin using Delegates for the logger. In this case, we will be creating the logger using the lazy keyword. This way, we will create the object only when we access it. Delegates are a great way to postpone object creation until we use it. This improves startup time (which is much needed and appreciated in Android). So let us explore a method using lazy delegates in Kotlin:
- We'll use java.util.Logging internally, but this works for any Logging library of your choice. So let’s use the Kotlin’s lazy delegate to get our logger:
public fun <R : Any> R.logger(): Lazy<Logger> {
return lazy { Logger.getLogger(this.javaClass.name) }
}
- Now in our class, we can simply call the method to get our logger and use it:
class SomeClass {
companion object { val log by logger() }
fun do_something() {
log.info("Did Something")
}
}
When you run the code, you can see the following output:
Sep 25, 2017 10:49:00 PM packageA.SomeClass do_something
INFO: Did Something
So, as we can see in the output, we get the class name and method name too (if you are accessing logger inside a method).