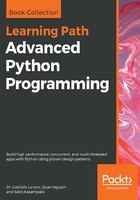
Sharing declarations
When writing your Cython modules, you may want to reorganize your most used functions and classes declaration in a separate file so that they can be reused in different modules. Cython allows you to put these components in a definition file and access them with cimport statements.
Let's say that we have a module with the max and min functions, and we want to reuse those functions in multiple Cython programs. If we simply write a bunch of functions in a .pyx file, the declarations will be confined to the same file.
To share the max and min functions, we need to write a definition file with a .pxd extension. Such a file only contains the types and function prototypes that we want to share with other modules--a public interface. We can declare the prototypes of our max and min functions in a file named mathlib.pxd, as follows:
cdef int max(int a, int b)
cdef int min(int a, int b)
As you can see, we only write the function name and arguments without implementing the function body.
The function implementation goes into the implementation file with the same base name but the .pyx extension--mathlib.pyx:
cdef int max(int a, int b):
return a if a > b else b
cdef int min(int a, int b):
return a if a < b else b
The mathlib module is now importable from another Cython module.
To test our new Cython module, we will create a file named distance.pyx containing a function named chebyshev. The function will calculate the Chebyshev distance between two points, as shown in the following code. The Chebyshev distance between two coordinates--(x1, y1) and (x2, y2)--is defined as the maximum value of the difference between each coordinate:
max(abs(x1 - x2), abs(y1 - y2))
To implement the chebyshev function, we will use the max function declared in mathlib.pxd by importing it with the cimport statement, as shown in the following code snippet:
from mathlib cimport max
def chebyshev(int x1, int y1, int x2, int y2):
return max(abs(x1 - x2), abs(y1 - y2))
The cimport statement will read mathlib.pxd and the max definition will be used to generate the distance.c file